In my previous post I demonstrated how one can easily render data on the client side from the Categories table in the Northwind database using KnockoutJS. This post is a slight variation of my previous post whereby the source of the data is an oData feed originating from http://services.odata.org/Northwind/Northwind.svc.
Pre-requisites:
- Visual Studio 2010
- MVC 3.0
- NuGet
- KnockoutJS
- DataJS
Step 1:
Start a new New Web Site project in Visual Studio 2010 using the ASP.NET Empty Web Site template.
Step 2:
In Solution Explorer, right-click on the top node and select “Manage NuGet Packages …”.
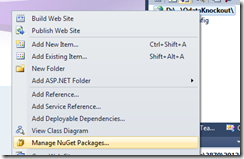
Step 3:
Enter “knockout” in the search field on the top right-hand-side of the next screen. Select “knockoutjs” then click on the “Install” button.
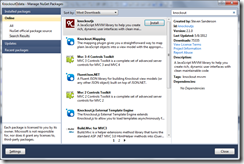
Step 4:
In addition to knockoutjs, we will be using other JavaScript libraries jQuery and DataJS. DataJS facilitates access to oData. Therefore, repeat Step-3 for jQuery and DataJs libraries.

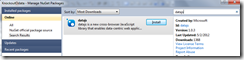
Ensure that the jQuery, DataJs and KnockoutJs libraries are installed into your project under the Scripts folder as shown below:
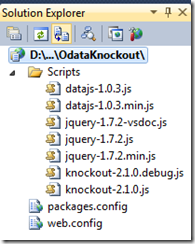
Step 5:
Add a new page named “Default.htm” to your website.
Open the Default.htm file. Drag and drop the JavaScript files jquery-1.7.2.js, knockout-2.1.0.js and datajs-1.0.3.js from the Scripts folder into the Default.htm file just below the ending </title> tag. This produces the <script> tag pointing to the respective JavaScript files. The page should resemble the following:
<!DOCTYPE>
<html>
<head>
<title></title>
<script src="Scripts/jquery-1.7.2.js" type="text/javascript"></script>
<script src="Scripts/knockout-2.1.0.js" type="text/javascript"></script>
<script src="Scripts/datajs-1.0.3.js" type="text/javascript"></script>
</head>
<body>
</body>
</html>
Step 6:
Place the following HTML into the body section of the Default.htm file:
<ul data-bind="foreach: categories">
<li><span data-bind="text: $data.CategoryName"></span></li>
</ul>
<script type="text/javascript">
var ServiceURL = "http://services.odata.org/Northwind/Northwind.svc";
OData.read(ServiceURL + "/Categories?$format=json", CallbackFunction);
function CallbackFunction(data, request) {
var viewModel = {
categories: data.results
};
ko.applyBindings(viewModel);
}
</script>
Let’s analyze the above code. The most important line is:
OData.read(url, CallbackFunction);
The OData.read() function comes from the “DataJS” library. Its first argument is an oData URL, and the second argument is a callback function that is called once a response is received from the server. Notice that the oData URL contains “$format=json” so that data returned from the service is in JSON format.
The callback function reads the JSON data encapsulated in property data.results into the categories property of the KnockoutJS viewModel. Finally, the viewModel is bound to the view with the function call ko.applyBindings(viewModel).
On the view side, data is rendered to the web page as follows:
<ul data-bind="foreach: categories">
<li><span data-bind="text: $data.CategoryName"></span></li>
</ul>
The final state of the web page is:
<!DOCTYPE>
<html>
<head>
<title></title>
<script src="Scripts/jquery-1.7.2.js" type="text/javascript"></script>
<script src="Scripts/knockout-2.1.0.js" type="text/javascript"></script>
<script src="Scripts/datajs-1.0.3.js" type="text/javascript"></script>
</head>
<body>
<ul data-bind="foreach: categories">
<li><span data-bind="text: $data.CategoryName"></span></li>
</ul>
<script type="text/javascript">
var ServiceURL = "http://services.odata.org/Northwind/Northwind.svc";
OData.read(ServiceURL + "/Categories?$format=json", CallbackFunction);
function CallbackFunction(data, request) {
var viewModel = {
categories: data.results
};
ko.applyBindings(viewModel);
}
</script>
</body>
</html>
This example, of course, a very simplistic. It does, however, illustrate how the various pieces fit together involving KnockoutJS and oData.
Hit F5 in Visual Studio 2010.
You may receive a browser warning because a request is being made to another server at http://www.odata.org. I received the following dialog, and clicked on the “Yes” button:
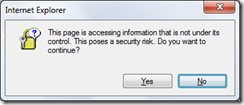
The output is identical to my previous post. The difference, this time, is that we are using plain HTML and all data access is being done using client-side technologies.
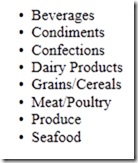